Flow Production Tracking Menus
Introduction
The Flow Production Tracking Menus class provide a standardized interface, look and feel for building menus that interact with Flow Production Tracking.
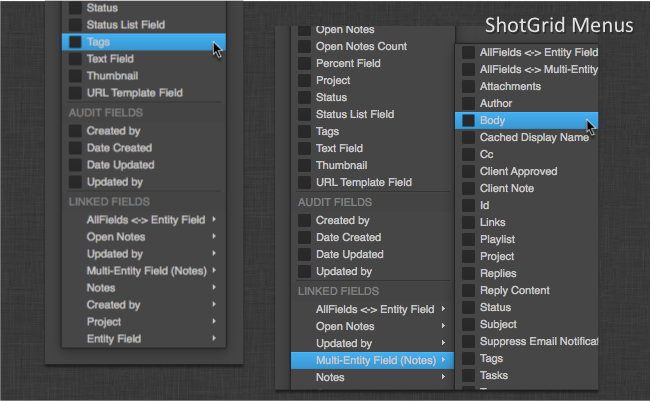
Flow Production Tracking Menu
- class shotgun_menus.ShotgunMenu(parent=None)[source]
Bases:
QMenu
A base class with support for easily adding labels and groups of actions with a consistent styling.
Usage Example:
shotgun_menus = sgtk.platform.import_framework("tk-framework-qtwidgets", "shotgun_menus") ShotgunMenu = shotgun_menus.ShotgunMenu # ... action1 = QtGui.QAction("Action 1", self) action2 = QtGui.QAction("Action 2", self) submenu = QtGui.QMenu("Submenu", self) menu = ShotgunMenu(self) menu.add_group([action1, action2, submenu], "My Actions")
Image shows the results of the
ShotgunMenu
created in the example.Initialize the menu.
- Parameters:
parent (
QWidget
) – The menu’s parent.
- add_group(items, title=None, separator=True, exclusive=False)[source]
Adds a group of items to the menu.
The list of items can include
QAction
orQMenu
instances. If atitle
is supplied, a non-clickable label will be added with the supplied text at the top of the list of items in the menu. By default, a separator will be added above the group unlessFalse
is supplied for the optionalseparator
argument. A separator will not be included if the group is added to an empty menu.A list of all actions, including separator, label, and menu actions, in the order added, will be returned.
- Parameters:
items (list) – A list of actions and/or menus to add to this menu.
title (str) – Optional text to use in a label at the top of the group.
separator (bool) – Add a separator if
True
(default), don’t add ifFalse
.exclusive (bool) – If exclusive is set to
True
, the added items will be an exclusive group. If the items are checkable, only one will be checkable at any given time. The default isFalse
.
- Returns:
A list of added
QAction
objects- Return type:
Entity Field Menu
- class shotgun_menus.EntityFieldMenu(sg_entity_type, parent=None, bg_task_manager=None, project_id=None)[source]
Bases:
ShotgunMenu
A menu that automatically displays the fields for a given Shotgun entity.
- The QActions for the menu will all have their data set to a dictionary in the form:
{“field”: selected_field}
Constructor
- Parameters:
sg_entity_type (String) – The entity type to build a menu for
parent (
QWidget
) – Parent widgetbg_task_manager (
BackgroundTaskManager
) – The task manager the menu will use if it needs to run a taskproject_id (int) – The project Entity id. If None, the current context’s project will be used, or the “site” cache location will be returned if the current context does not have an associated project.
- set_field_filter(field_filter)[source]
Set the callback used to filter which fields are shown by the menu.
- Parameters:
field_filter (A method that takes a single field string as its only argument and returns a boolean) – Callback called for each entity field which returns True if the field should be shown and False if it should not. The fields will be in “bubbled” notation, for example “sg_sequence.Sequence.code”
- set_checked_filter(checked_filter)[source]
Set the callback used to set which fields are checked. By specifying a value other than None, all the menu items will be checkable.
- Parameters:
checked_filter (A method that takes a single field string as its only argument and returns a boolean) – Callback called for each entity field which returns True if the field should be checked and False if it should not. The fields will be in “bubbled” notation, for example “sg_sequence.Sequence.code”
- set_disabled_filter(disabled_filter)[source]
Set the callback used to filter which fields are disabled
- Parameters:
disabled_filter (A method that takes a single field string as its only argument and returns a boolean) – Callback called for each entity field which returns True if the field should be disabled and False if it should not. The fields will be in “bubbled” notation, for example “sg_sequence.Sequence.code”
- set_entity_type_filter(entity_type_filter)[source]
Set the callback used to filter what entity types to display in submenus
- Parameters:
entity_type_filter (A method that takes a single entity types string as its only argument and returns a boolean) – Callback called for each entity type which returns True if the given entity type should be displayed