Descriptors
Descriptors are abstractions used to describe a remote piece of code or configuration. They handle caching of remote resources locally, making it easy to write workflows around installation and deployment.
Note
The Toolkit descriptor API does not require an installed or
bootstrapped instance of the Toolkit Core platform. You can use it
simply by importing sgtk
and calling its methods.
Descriptors are used extensively by Toolkit and allow a user to configure and drive Toolkit in a flexible fashion. Descriptors typically point at a remote location and makes it easy to handle code transport from that location into a local cache. Descriptors form the backbone for Flow Production Tracking deployment and installation. The following example shows basic usage:
import sgtk
from sgtk.descriptor import Descriptor
# first, authenticate our user
sg_auth = sgtk.authentication.ShotgunAuthenticator()
user = sg_auth.get_user()
sg = user.create_sg_connection()
# we are fetching v1.2.3 of the default config from the app store
uri = "sgtk:descriptor:app_store?name=tk-config-basic&version=v1.2.3"
# create object
desc = sgtk.descriptor.create_descriptor(sg, Descriptor.CONFIG, uri)
# make sure this is cached locally on disk
desc.ensure_local()
# check if it is the latest version
latest_desc = desc.find_latest_version()
# see what core is needed for this config
required_core_uri = desc.associated_core_descriptor
When descriptors serialized, they can have two different forms:
A descriptor URI is a compact string representation, for example
sgtk:descriptor:app_store?name=tk-config-default&version=v1.2.3
A descriptor dictionary contains the equivalent information in key-value pair form:
{ "type": "app_store", "version": "v1.2.3", "name": "tk-config-default" }
Note
To convert between descriptor URI format and dictionary format, you can use
the methods descriptor_dict_to_uri()
and descriptor_uri_to_dict()
.
When a the payload of a descriptor is downloaded, it ends up by default in the global bundle cache.
Note
The global bundle cache can be found in the following locations:
Macosx:
~/Library/Caches/Shotgun/bundle_cache
Windows:
%APPDATA%\Shotgun\Caches\bundle_cache
Linux:
~/.shotgun/caches/bundle_cache
Unless specified otherwise, this is the location where descriptors will be cached locally.
Older versions of Toolkit Core (prior to v0.18) used to maintain a bundle cache
in an install
folder inside the pipeline configuration location.
In addition to the location above, you can specify additional folders where the descriptor API will go and look for cached items. This is useful if you want to pre-cache an environment for your studio or distribute a set of app and engine versions as an installable package that require no further retrieval in order to function.
Alternatively, you can set the SHOTGUN_BUNDLE_CACHE_PATH
environment variable to
a cache path on disk. This override helps facilitate workflows that require a
centralized disk location to which the descriptors are cached.
Descriptor types
Several different descriptor types are supported by Toolkit:
An app_store descriptor represents an item in the Toolkit App Store
A shotgun descriptor represents an item stored in Flow Production Tracking
A git descriptor represents a tag in a git repository
A git_branch descriptor represents a commit in a git branch
A github_release descriptor represents a Release on a Github repo
A path descriptor represents a location on disk
A dev descriptor represents a developer sandbox
A manual descriptor gives raw access to the bundle caching structure
The descriptor API knows how to access and locally cache each of the types above. You can control the location where the API caches items and supply additional lookup locations if you want to pre-bake your own collection of caches. Descriptors that are downloaded (cached) to the local disk are called downloadable descriptors. The app_store, shotgun, git and git_branch descriptors are downloadable descriptors, while the path, dev and manual descriptors are accessed directly from the specified path.
The Flow Production Tracking App store
The Flow Production Tracking app store is used to release and distribute versions of Apps, Engines, Configs etc. that have been tested and approved by Flow Production Tracking. App store descriptors should include a name and version token and are on the following form:
{
type: app_store,
name: tk-core,
version: v12.3.4
}
sgtk:descriptor:app_store?name=tk-core&version=v12.3.4
App store may also have an optional label
parameter. This indicates that the descriptor is tracking
against a particular label in the app store and will not download updates which do not have the label assigned:
{
type: app_store,
name: tk-core,
version: v12.3.4,
label: v2018.3
}
sgtk:descriptor:app_store?name=tk-core&version=v12.3.4&label=v2018.3
A label can for example be used by plugins that are bundled with DCCs to only receive app store updates
targeting that particular DCC version. If you for example are running a plugin bundled together with
v2017 of a DCC, the plugin can be set up to track against the latest released version of
sgtk:descriptor:app_store?name=tk-config-dcc&label=v2017
. In this case, when the descriptor is checking
for the latest available version in the app store, only versions labelled with v2017 will be taken into account.
Tracking against commits in a git branch
The git_branch
descriptor type is typically used during development and allows you to track
a commit in a particular branch.
Git repository residing on the local file system:
{
type: git_branch,
branch: master,
path: /path/to/repo.git,
version: 17fedd8
}
sgtk:descriptor:git_branch?branch=master&path=/path/to/repo.git&version=17fedd8
SSH git syntax:
{
type: git_branch,
branch: master,
path: user@remotehost:/path_to/repo.git,
version: 17fedd8
}
sgtk:descriptor:git_branch?branch=master&path=user@remotehost:/path_to/repo.git&version=17fedd8
Git protocol syntax:
{
type: git_branch,
branch: master,
path: git://github.com/user/tk-multi-publish.git,
version: 17fedd8
}
sgtk:descriptor:git_branch?branch=master&path=git://github.com/user/tk-multi-publish.git&version=17fedd8
Http protocol syntax:
{
type: git_branch,
branch: master,
path: https://github.com/user/tk-multi-publish.git,
version: 17fedd8
}
sgtk:descriptor:git_branch?branch=master&path=https://github.com/user/tk-multi-publish.git&version=17fedd8
You can use both long and short hash formats for the version token. The latest version for a git_branch descriptor is defined as the most recent commit for a given branch.
Warning
Repositories requiring authentication are not fully supported by Toolkit. For such
setups, we strongly recommend using an ssh style git url
(e.g. git@github.com:shotgunsoftware/tk-core.git
) in order to eliminate git
trying to prompt for a password in the background.
Note
On Windows, it is recommended that you use forward slashes.
Note
When using the git descriptor, you need to have the git executable in
the PATH
in order for the API to be able to do a latest check or
app download. The git executable is, however, not needed during descriptor
resolve and normal operation.
Tracking against releases on Github
The github_release
descriptor type is useful for studios and 3rd parties wishing to deploy apps directly from Github.
Getting tk-multi-pythonconsole
from its shotgunsoftware
Github repo:
{
type: github_release,
organization: shotgunsoftware,
repository: tk-multi-pythonconsole,
version: v1.2.29
}
sgtk:descriptor:github_release?organization=shotgunsoftware&repository=tk-multi-pythonconsole&version=v1.2.29
organization
is the Github organization or user that the repository belongs to.repository
is the name of the repository to find a Release for.version
is the name of the Release to use.private
is an optional setting that defines whether the repository is private and requires authentication.
Private repositories are supported through the use of the Github api, authenticated with personal access tokens. A token must be set as environment variable that is specific to the organization setting:
SG_GITHUB_TOKEN_<ORGANIZATION>
defines the personal access token used to authenticate. The organization name should be uppercase and snake cased.
Note
This descriptor only works with Github Releases, not all tags. For more information, see the Github Documentation on Releases.
Note
If you want constraint patterns (i.e. v1.x.x
) to work correctly with this descriptor, you must follow the semantic versioning specification when naming Releases on Github.
Note
For private repos, it’s recommended that you use a fine-grained personal access token with read-only access to Content. For more information, see the Github Documentation on Personal Access Tokens.
Pointing to a path on disk
Pointing Toolkit to an app that resides in the local file system is often very useful for managing your own bundles
or doing development on an app or engine before releasing onto production. To allow for these scenarios, Toolkit
provides the dev
and path
descriptors.
Note
dev
and path
descriptors are using the exact same syntax. The difference between them is that
the dev
descriptor indicates that development is happening and Toolkit will use this to enable developer
related functionality at runtime, such as making available options to automatically reload the code at runtime.
For production deploys, always use the path
descriptor.
Basic example with a path intended to be used on the local operating system - the typical setup for doing personal development:
{
type: dev,
path: /path/to/app
}
sgtk:descriptor:dev?path=/path/to/app
When using a path
descriptor in production, you can include paths to multiple different operating systems:
{
type: path,
windows_path: c:\path\to\app,
linux_path: /path/to/app,
mac_path: /path/to/app
}
sgtk:descriptor:path?linux_path=/path/to/app&mac_path=/path/to/app&windows_path=c:\path\to\app
Environment variables can be included in paths, they will be expanded using os.path.expandvars()
and os.path.expanduser()
.
This is only supported in dev
and path
descriptors.
{
type: dev,
path: ${HOME}/path/to/app
}
sgtk:descriptor:dev?path=%24%7BHOME%7D/path/to/app
Home directory ~ syntax will be expanded:
{
type: path,
path: ~/path/to/app
}
sgtk:descriptor:path?path=%7E/path/to/app
Note
Special characters are escaped for uri string compatibility
Sometimes it may be useful to distribute application code within the configuration for applications
that may not be accessible through the traditional distribution mechanism for Toolkit applications
like the App Store or the various Git
descriptor types. Although we recommend in those case to use the local bundle
caches, it may not always be desirable. In those cases, it is possible to
package the application code alongside the env
or hooks
folder and refer to it with the
CONFIG_FOLDER
token:
{
type: dev,
path: {CONFIG_FOLDER}/dev/tk-nuke-myapp
}
sgtk:descriptor:dev?path=%7BCONFIG_FOLDER%7D/dev/tk-nuke-myapp
When using an installed pipeline configuration, this will refer to
{PIPELINE_ROOT}/config
. When using a cached or baked pipeline configuration, it will point where
the bundle was found in one of the bundle caches.
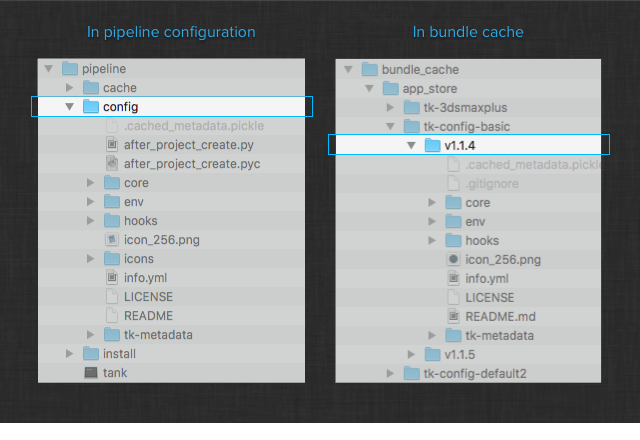
Note
If you don’t know if your configuration will be used with an installed, baked or cached pipeline
configuration, we recommend the use of the CONFIG_FOLDER
token as it allows to resolve where
the configuration’s files will be located as the pipeline configuration may or may not contain
the config
folder depending on usage.
When using an installed pipeline configuration, it can be useful to organize your development
sandbox relative to a pipeline configuration. If all developers in the studio share a convention
where they for example have a dev
folder inside their pipeline configuration dev sandboxes, it
becomes easy to exchange environment configs. You can achieve this by using the special token
{PIPELINE_CONFIG}
which will resolve into the local path to the pipeline configuration:
{
type: dev,
path: {PIPELINE_CONFIG}/dev/tk-nuke-myapp
}
sgtk:descriptor:dev?path=%7BPIPELINE_CONFIG%7D/dev/tk-nuke-myapp
Note
The PIPELINE_CONFIG
refers to the root of the pipeline configuration, which for cached or baked
pipeline configurations do not contain the config
folder with all the environment and hook files.
If you wish to refer to elements inside the config
folder and wish the configuration to be usable
both with installed, baked or cached configuration, we recommend you use the CONFIG_FOLDER
token.
Pointing at a file attachment in Flow Production Tracking
The Shotgun descriptor allows you to upload an attachment directly to Flow Production Tracking and then reference it with a descriptor.
This allows for workflows where you can distribute configurations, custom apps or other items to your distributed users - regardless of network or file access. All they need is a connection to Flow Production Tracking.
A practical application of this is Toolkit’s cloud based configurations;
Upload a zipped toolkit configuration to the PipelineConfiguration.uploaded_config
field on your pipeline configuration.
The ToolkitManager
bootstrapping interface will automatically detect
this, download the configuration locally and use this when launching.
This allows for a powerful workflow where a configuration is simply
uploaded to Flow Production Tracking and it gets automatically picked up by all
users (even if they are remote).
The Shotgun descriptor is the low level mechanism that is used to implement the cloud configurations described above. The descriptor points at a particular attachment field in Flow Production Tracking and expects a zip file to be uploaded to the field.
Two formats are supported, one explicit based on a shotgun entity id and
one implicit which uses the name in shotgun to resolve a record. With the
id based syntax you specify the Flow Production Tracking entity type and field name you want
to look for and the entity id to inspect. For example, if your attachment field is called
PipelineConfiguration.uploaded_config
and you want to access the uploaded payload for
the Pipeline Configuration entity with id 111, use the following descriptor:
{
type: shotgun,
entity_type: PipelineConfiguration, # entity type
id: 111, # shotgun entity id
field: uploaded_config, # attachment field where payload can be found
version: 222 # attachment id of particular attachment
}
sgtk:descriptor:shotgun?entity_type=PipelineConfiguration&id=111&field=sg_config&version=222
The version token above refers to the version of the attachment. Every time a new attachment is uploaded to Flow Production Tracking, it gets assigned a unique id and the version number in the descriptor allows you to point at a particular version of an uploaded attachment. It is also used to handle the underlying logic to understand what the latest version of an attachment is.
In some workflows, typically where you follow name based naming conventions, the following syntax can be useful:
{
type: shotgun,
entity_type: PipelineConfiguration, # entity type
name: Primary, # name of the record in shotgun (e.g. 'code' field)
project_id: 123, # optional project id. If omitted, name is assumed to be unique.
field: uploaded_config, # attachment field where payload can be found
version: 456 # attachment id of particular attachment
}
sgtk:descriptor:shotgun?entity_type=PipelineConfiguration&name=primary&project_id=123&field=sg_config&version=456
Here, instead of specifying the entity id you can specify a name
and an optional project_id
field. The name
field will be translated into an appropriate Flow Production Tracking name field, typically the code
field.
Manual Descriptors
Toolkit also provides a manual
mode to make it easy to manage production installations of apps
and engines without any automation. When you use the manual descriptor, it is up to you to install the code in the right
location and no automated update checks will ever take place. The manual mode uses the following syntax:
{
type: manual,
name: tk-nuke-publish,
version: v0.5.0
}
sgtk:descriptor:manual?name=tk-nuke-publish&version=v0.5.0
It will look for the code in a manual
folder in the bundle cache, so with the example above, Toolkit would look
for the code in the BUNDLE_CACHE/manual/tk-nuke-publish/v0.5.0
folder.
Warning
Manual descriptors are part of an older toolkit workflow methodology and while they are supported, we do not recommend using them.
Environment Variables
A number of different environment variables exist to help control the behavior of Descriptors.
API reference
Factory Methods
- sgtk.descriptor.create_descriptor(sg_connection, descriptor_type, dict_or_uri, bundle_cache_root_override=None, fallback_roots=None, resolve_latest=False, constraint_pattern=None, local_fallback_when_disconnected=True)[source]
Factory method. Use this when creating descriptor objects.
Note
Descriptors inherit their threading characteristics from the shotgun connection that they carry internally. They are reentrant and should not be passed between threads.
- Parameters:
sg_connection – Shotgun connection to associated site
descriptor_type – Either
Descriptor.APP
,CORE
,CONFIG
,INSTALLED_CONFIG
,ENGINE
orFRAMEWORK
dict_or_uri – A std descriptor dictionary dictionary or string
bundle_cache_root_override – Optional override for root path to where downloaded apps are cached. If not specified, the global bundle cache location will be used. This location is a per-user cache that is shared across all sites and projects.
fallback_roots – Optional List of immutable fallback cache locations where apps will be searched for. Note that when descriptors download new content, it always ends up in the bundle_cache_root.
resolve_latest –
If true, the latest version will be determined and returned.
If set to True, no version information needs to be supplied with the descriptor dictionary/uri for descriptor types which support a version number concept. Please note that setting this flag to true will typically affect performance - an external connection is often required in order to establish what the latest version is.
If a remote connection cannot be established when attempting to determine the latest version, a local scan will be carried out and the highest version number that is cached locally will be returned.
constraint_pattern –
If resolve_latest is True, this pattern can be used to constrain the search for latest to only take part over a subset of versions. This is a string that can be on the following form:
v0.1.2
,v0.12.3.2
,v0.1.3beta
- a specific versionv0.12.x
- get the highest v0.12 versionv1.x.x
- get the highest v1 version
local_fallback_when_disconnected – If resolve_latest is set to True, specify the behaviour in the case when no connection to a remote descriptor can be established, for example because and internet connection isn’t available. If True, the descriptor factory will attempt to fall back on any existing locally cached bundles and return the latest one available. If False, a
TankDescriptorError
is raised instead.
- Returns:
Descriptor
object- Raises:
- sgtk.descriptor.descriptor_dict_to_uri(ddict)[source]
Translates a descriptor dictionary into a uri.
- Parameters:
ddict – descriptor dictionary
- Returns:
descriptor uri
- sgtk.descriptor.descriptor_uri_to_dict(uri)[source]
Translates a descriptor uri into a dictionary.
- Parameters:
uri – descriptor string uri
- Returns:
descriptor dictionary
- sgtk.descriptor.is_descriptor_version_missing(dict_or_uri)[source]
Helper method which checks if a descriptor needs a version.
If the given descriptor dictionary or uri is one of the types which requires a version token, and this token is not defined,
True
will be returned, otherwiseFalse
.This is useful for a standard pattern which can be used used where you want to allow users to configure toolkit descriptors which track either the latest version or a specific one. In this pattern, the user hints that they want to track latest version by omitting the version token altogether.
The following standard pattern can then be implemented:
# determine if we should request the latest version # of the given descriptor if is_descriptor_version_missing(descriptor_uri): # require the descriptor system to return # the latest descriptor it can detect resolve_latest = True else: # normal direct lookup of a particular # descriptor version resolve_latest = False descriptor_obj = create_descriptor( sg_connection, Descriptor.CONFIG, descriptor_uri, resolve_latest=resolve_latest )
- Parameters:
dict_or_uri – A std descriptor dictionary dictionary or string
- Returns:
Boolean to indicate if a required version token is missing
AppDescriptor
- class sgtk.descriptor.AppDescriptor(sg_connection, io_descriptor, bundle_cache_root_override, fallback_roots)[source]
Descriptor that describes a Toolkit App
Note
Use the factory method
create_descriptor()
when creating new descriptor objects.- Parameters:
sg_connection – Connection to the current site.
io_descriptor – Associated IO descriptor.
bundle_cache_root_override – Override for root path to where downloaded apps are cached.
fallback_roots – List of immutable fallback cache locations where apps will be searched for.
- property changelog
Information about the changelog for this item.
- Returns:
A tuple (changelog_summary, changelog_url). Values may be
None
to indicate that no changelog exists.
- check_version_constraints(core_version=None, engine_descriptor=None, desktop_version=None)
Checks if there are constraints blocking an upgrade or install.
- Parameters:
- Raises:
Raised if one or multiple constraint checks has failed.
- Return type:
sgtk.descriptor.CheckVersionConstraintsError
- clone_cache(cache_root)
The descriptor system maintains an internal cache where it downloads the payload that is associated with the descriptor. Toolkit supports complex cache setups, where you can specify a series of path where toolkit should go and look for cached items.
This is an advanced method that helps in cases where a user wishes to administer such a setup, allowing a cached payload to be copied from its current location into a new cache structure.
If the descriptor’s payload doesn’t exist on disk, it will be downloaded.
- Parameters:
cache_root – Root point of the cache location to copy to.
- property configuration_schema
The manifest configuration schema for this bundle. Always returns a dictionary.
- Returns:
Configuration dictionary as defined in the manifest or {} if not defined
- copy(target_folder)
Copy the config descriptor into the specified target location.
- Parameters:
target_folder – Folder to copy the descriptor to
- property deprecation_status
Information about deprecation status.
- Returns:
Returns a tuple (is_deprecated, message) to indicate if this item is deprecated.
- property description
A short description of the item.
- property display_name
The display name for this item. If no display name has been defined, the system name will be returned.
- property documentation_url
The documentation url for this item. If no documentation url has been defined, a url to the toolkit user guide is returned.
- download_local()
Retrieves this version to local repo.
- ensure_local()
Helper method. Ensures that the item is locally available.
- ensure_shotgun_fields_exist(tk=None)
Ensures that any shotgun fields a particular descriptor requires exists in shotgun. In the metadata (
info.yml
) for an app or an engine, it is possible to define a section for this:# the Shotgun fields that this app needs in order to operate correctly requires_shotgun_fields: Version: - { "system_name": "sg_movie_type", "type": "text" }
This method will retrieve the metadata and ensure that any required fields exists.
Warning
This feature may be deprecated in the future.
- Parameters:
tk – Core API instance to use for post install execution. This value defaults to
None
for backwards compatibility reasons and in the case a None value is passed in, the hook will not execute.
- exists_local()
Returns true if this item exists in a local repo.
- find_latest_cached_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version that can be found in the local bundle caches.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
Instance derived from
Descriptor
orNone
if no cached version is available.
- find_latest_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
instance derived from
Descriptor
- get_dict()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_location()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_path()
Returns the path to a location where this item is cached.
When locating the item, any bundle cache fallback paths will first be searched in the order they have been defined, and lastly the main bundle cached will be checked.
If the item is not locally cached,
None
is returned.- Returns:
Path string or
None
if not cached.
- get_uri()
Returns the uri associated with this descriptor The uri is a string based representation that is equivalent to the descriptor dictionary returned by the get_dict() method.
- Returns:
Uri string that can be used to construct the descriptor
- has_remote_access()
Probes if the current descriptor is able to handle remote requests. If this method returns, true, operations such as
download_local()
andfind_latest_version()
can be expected to succeed.- Returns:
True if a remote is accessible, false if not.
- property icon_256
The path to a 256px square png icon file representing this item
- is_dev()
Returns true if this item is intended for development purposes
- Returns:
True if this is a developer item
- is_immutable()
Returns true if this descriptor never changes its content. This is true for most descriptors as they represent a particular version, tag or commit of an item. Examples of non-immutable descriptors include path and dev descriptors, where the descriptor points at a “live” location on disk where a user can make changes at any time.
- Returns:
True if this is a developer item
- property required_context
The required context, if there is one defined, for a bundle. This is a list of strings, something along the lines of [“user”, “task”, “step”] for an app that requires a context with user task and step defined.
- Returns:
A list of strings, with an empty list meaning no items required.
- property required_frameworks
A list of required frameworks for this item.
Always returns a list - for example:
[{'version': 'v0.1.0', 'name': 'tk-framework-widget'}]
Each item contains a name and a version key.
- Returns:
list of dictionaries
- run_post_install(tk=None)
If a post install hook exists in a descriptor, execute it. In the hooks directory for an app or engine, if a ‘post_install.py’ hook exists, the hook will be executed upon each installation.
Errors reported in the post install hook will be reported to the error log but execution will continue.
Warning
We no longer recommend using post install hooks. Should you need to use one, take great care when designing it so that it can execute correctly for all users, regardless of their shotgun and system permissions.
- Parameters:
tk – Core API instance to use for post install execution. This value defaults to
None
for backwards compatibility reasons and in the case a None value is passed in, the hook will not execute.
- property support_url
A url that points at a support web page associated with this item. If not url has been defined,
None
is returned.
- property supported_engines
The engines supported by this app or framework. Examples of return values:
None
- Works in all engines.["tk-maya", "tk-nuke"]
- Works in Maya and Nuke.
- property supported_platforms
The platforms supported. Possible values are windows, linux and mac.
Always returns a list, returns an empty list if there is no constraint in place.
example: [“windows”, “linux”] example: []
- property system_name
A short name, suitable for use in configuration files and for folders on disk.
- property version
The version number string for this item.
- property version_constraints
A dictionary with version constraints. The absence of a key indicates that there is no defined constraint. The following keys can be returned: min_sg, min_core, min_engine and min_desktop
- Returns:
Dictionary with optional keys min_sg, min_core, min_engine and min_desktop
EngineDescriptor
- class sgtk.descriptor.EngineDescriptor(sg_connection, io_descriptor, bundle_cache_root_override, fallback_roots)[source]
Descriptor that describes a Toolkit Engine
Note
Use the factory method
create_descriptor()
when creating new descriptor objects.- Parameters:
sg_connection – Connection to the current site.
io_descriptor – Associated IO descriptor.
bundle_cache_root_override – Override for root path to where downloaded apps are cached.
fallback_roots – List of immutable fallback cache locations where apps will be searched for.
- property changelog
Information about the changelog for this item.
- Returns:
A tuple (changelog_summary, changelog_url). Values may be
None
to indicate that no changelog exists.
- check_version_constraints(core_version=None, engine_descriptor=None, desktop_version=None)
Checks if there are constraints blocking an upgrade or install.
- Parameters:
- Raises:
Raised if one or multiple constraint checks has failed.
- Return type:
sgtk.descriptor.CheckVersionConstraintsError
- clone_cache(cache_root)
The descriptor system maintains an internal cache where it downloads the payload that is associated with the descriptor. Toolkit supports complex cache setups, where you can specify a series of path where toolkit should go and look for cached items.
This is an advanced method that helps in cases where a user wishes to administer such a setup, allowing a cached payload to be copied from its current location into a new cache structure.
If the descriptor’s payload doesn’t exist on disk, it will be downloaded.
- Parameters:
cache_root – Root point of the cache location to copy to.
- property configuration_schema
The manifest configuration schema for this bundle. Always returns a dictionary.
- Returns:
Configuration dictionary as defined in the manifest or {} if not defined
- copy(target_folder)
Copy the config descriptor into the specified target location.
- Parameters:
target_folder – Folder to copy the descriptor to
- property deprecation_status
Information about deprecation status.
- Returns:
Returns a tuple (is_deprecated, message) to indicate if this item is deprecated.
- property description
A short description of the item.
- property display_name
The display name for this item. If no display name has been defined, the system name will be returned.
- property documentation_url
The documentation url for this item. If no documentation url has been defined, a url to the toolkit user guide is returned.
- download_local()
Retrieves this version to local repo.
- ensure_local()
Helper method. Ensures that the item is locally available.
- ensure_shotgun_fields_exist(tk=None)
Ensures that any shotgun fields a particular descriptor requires exists in shotgun. In the metadata (
info.yml
) for an app or an engine, it is possible to define a section for this:# the Shotgun fields that this app needs in order to operate correctly requires_shotgun_fields: Version: - { "system_name": "sg_movie_type", "type": "text" }
This method will retrieve the metadata and ensure that any required fields exists.
Warning
This feature may be deprecated in the future.
- Parameters:
tk – Core API instance to use for post install execution. This value defaults to
None
for backwards compatibility reasons and in the case a None value is passed in, the hook will not execute.
- exists_local()
Returns true if this item exists in a local repo.
- find_latest_cached_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version that can be found in the local bundle caches.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
Instance derived from
Descriptor
orNone
if no cached version is available.
- find_latest_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
instance derived from
Descriptor
- get_dict()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_location()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_path()
Returns the path to a location where this item is cached.
When locating the item, any bundle cache fallback paths will first be searched in the order they have been defined, and lastly the main bundle cached will be checked.
If the item is not locally cached,
None
is returned.- Returns:
Path string or
None
if not cached.
- get_uri()
Returns the uri associated with this descriptor The uri is a string based representation that is equivalent to the descriptor dictionary returned by the get_dict() method.
- Returns:
Uri string that can be used to construct the descriptor
- has_remote_access()
Probes if the current descriptor is able to handle remote requests. If this method returns, true, operations such as
download_local()
andfind_latest_version()
can be expected to succeed.- Returns:
True if a remote is accessible, false if not.
- property icon_256
The path to a 256px square png icon file representing this item
- is_dev()
Returns true if this item is intended for development purposes
- Returns:
True if this is a developer item
- is_immutable()
Returns true if this descriptor never changes its content. This is true for most descriptors as they represent a particular version, tag or commit of an item. Examples of non-immutable descriptors include path and dev descriptors, where the descriptor points at a “live” location on disk where a user can make changes at any time.
- Returns:
True if this is a developer item
- property required_context
The required context, if there is one defined, for a bundle. This is a list of strings, something along the lines of [“user”, “task”, “step”] for an app that requires a context with user task and step defined.
- Returns:
A list of strings, with an empty list meaning no items required.
- property required_frameworks
A list of required frameworks for this item.
Always returns a list - for example:
[{'version': 'v0.1.0', 'name': 'tk-framework-widget'}]
Each item contains a name and a version key.
- Returns:
list of dictionaries
- run_post_install(tk=None)
If a post install hook exists in a descriptor, execute it. In the hooks directory for an app or engine, if a ‘post_install.py’ hook exists, the hook will be executed upon each installation.
Errors reported in the post install hook will be reported to the error log but execution will continue.
Warning
We no longer recommend using post install hooks. Should you need to use one, take great care when designing it so that it can execute correctly for all users, regardless of their shotgun and system permissions.
- Parameters:
tk – Core API instance to use for post install execution. This value defaults to
None
for backwards compatibility reasons and in the case a None value is passed in, the hook will not execute.
- property support_url
A url that points at a support web page associated with this item. If not url has been defined,
None
is returned.
- property supported_engines
The engines supported by this app or framework. Examples of return values:
None
- Works in all engines.["tk-maya", "tk-nuke"]
- Works in Maya and Nuke.
- property supported_platforms
The platforms supported. Possible values are windows, linux and mac.
Always returns a list, returns an empty list if there is no constraint in place.
example: [“windows”, “linux”] example: []
- property system_name
A short name, suitable for use in configuration files and for folders on disk.
- property version
The version number string for this item.
- property version_constraints
A dictionary with version constraints. The absence of a key indicates that there is no defined constraint. The following keys can be returned: min_sg, min_core, min_engine and min_desktop
- Returns:
Dictionary with optional keys min_sg, min_core, min_engine and min_desktop
FrameworkDescriptor
- class sgtk.descriptor.FrameworkDescriptor(sg_connection, io_descriptor, bundle_cache_root_override, fallback_roots)[source]
Descriptor that describes a Toolkit Framework
Note
Use the factory method
create_descriptor()
when creating new descriptor objects.- Parameters:
sg_connection – Connection to the current site.
io_descriptor – Associated IO descriptor.
bundle_cache_root_override – Override for root path to where downloaded apps are cached.
fallback_roots – List of immutable fallback cache locations where apps will be searched for.
Returns a boolean indicating whether the bundle is a shared framework. Shared frameworks only have a single instance per instance name in the current environment.
- Returns:
True if the framework is shared
- property changelog
Information about the changelog for this item.
- Returns:
A tuple (changelog_summary, changelog_url). Values may be
None
to indicate that no changelog exists.
- check_version_constraints(core_version=None, engine_descriptor=None, desktop_version=None)
Checks if there are constraints blocking an upgrade or install.
- Parameters:
- Raises:
Raised if one or multiple constraint checks has failed.
- Return type:
sgtk.descriptor.CheckVersionConstraintsError
- clone_cache(cache_root)
The descriptor system maintains an internal cache where it downloads the payload that is associated with the descriptor. Toolkit supports complex cache setups, where you can specify a series of path where toolkit should go and look for cached items.
This is an advanced method that helps in cases where a user wishes to administer such a setup, allowing a cached payload to be copied from its current location into a new cache structure.
If the descriptor’s payload doesn’t exist on disk, it will be downloaded.
- Parameters:
cache_root – Root point of the cache location to copy to.
- property configuration_schema
The manifest configuration schema for this bundle. Always returns a dictionary.
- Returns:
Configuration dictionary as defined in the manifest or {} if not defined
- copy(target_folder)
Copy the config descriptor into the specified target location.
- Parameters:
target_folder – Folder to copy the descriptor to
- property deprecation_status
Information about deprecation status.
- Returns:
Returns a tuple (is_deprecated, message) to indicate if this item is deprecated.
- property description
A short description of the item.
- property display_name
The display name for this item. If no display name has been defined, the system name will be returned.
- property documentation_url
The documentation url for this item. If no documentation url has been defined, a url to the toolkit user guide is returned.
- download_local()
Retrieves this version to local repo.
- ensure_local()
Helper method. Ensures that the item is locally available.
- ensure_shotgun_fields_exist(tk=None)
Ensures that any shotgun fields a particular descriptor requires exists in shotgun. In the metadata (
info.yml
) for an app or an engine, it is possible to define a section for this:# the Shotgun fields that this app needs in order to operate correctly requires_shotgun_fields: Version: - { "system_name": "sg_movie_type", "type": "text" }
This method will retrieve the metadata and ensure that any required fields exists.
Warning
This feature may be deprecated in the future.
- Parameters:
tk – Core API instance to use for post install execution. This value defaults to
None
for backwards compatibility reasons and in the case a None value is passed in, the hook will not execute.
- exists_local()
Returns true if this item exists in a local repo.
- find_latest_cached_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version that can be found in the local bundle caches.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
Instance derived from
Descriptor
orNone
if no cached version is available.
- find_latest_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
instance derived from
Descriptor
- get_dict()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_location()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_path()
Returns the path to a location where this item is cached.
When locating the item, any bundle cache fallback paths will first be searched in the order they have been defined, and lastly the main bundle cached will be checked.
If the item is not locally cached,
None
is returned.- Returns:
Path string or
None
if not cached.
- get_uri()
Returns the uri associated with this descriptor The uri is a string based representation that is equivalent to the descriptor dictionary returned by the get_dict() method.
- Returns:
Uri string that can be used to construct the descriptor
- has_remote_access()
Probes if the current descriptor is able to handle remote requests. If this method returns, true, operations such as
download_local()
andfind_latest_version()
can be expected to succeed.- Returns:
True if a remote is accessible, false if not.
- property icon_256
The path to a 256px square png icon file representing this item
- is_dev()
Returns true if this item is intended for development purposes
- Returns:
True if this is a developer item
- is_immutable()
Returns true if this descriptor never changes its content. This is true for most descriptors as they represent a particular version, tag or commit of an item. Examples of non-immutable descriptors include path and dev descriptors, where the descriptor points at a “live” location on disk where a user can make changes at any time.
- Returns:
True if this is a developer item
- property required_context
The required context, if there is one defined, for a bundle. This is a list of strings, something along the lines of [“user”, “task”, “step”] for an app that requires a context with user task and step defined.
- Returns:
A list of strings, with an empty list meaning no items required.
- property required_frameworks
A list of required frameworks for this item.
Always returns a list - for example:
[{'version': 'v0.1.0', 'name': 'tk-framework-widget'}]
Each item contains a name and a version key.
- Returns:
list of dictionaries
- run_post_install(tk=None)
If a post install hook exists in a descriptor, execute it. In the hooks directory for an app or engine, if a ‘post_install.py’ hook exists, the hook will be executed upon each installation.
Errors reported in the post install hook will be reported to the error log but execution will continue.
Warning
We no longer recommend using post install hooks. Should you need to use one, take great care when designing it so that it can execute correctly for all users, regardless of their shotgun and system permissions.
- Parameters:
tk – Core API instance to use for post install execution. This value defaults to
None
for backwards compatibility reasons and in the case a None value is passed in, the hook will not execute.
- property support_url
A url that points at a support web page associated with this item. If not url has been defined,
None
is returned.
- property supported_engines
The engines supported by this app or framework. Examples of return values:
None
- Works in all engines.["tk-maya", "tk-nuke"]
- Works in Maya and Nuke.
- property supported_platforms
The platforms supported. Possible values are windows, linux and mac.
Always returns a list, returns an empty list if there is no constraint in place.
example: [“windows”, “linux”] example: []
- property system_name
A short name, suitable for use in configuration files and for folders on disk.
- property version
The version number string for this item.
- property version_constraints
A dictionary with version constraints. The absence of a key indicates that there is no defined constraint. The following keys can be returned: min_sg, min_core, min_engine and min_desktop
- Returns:
Dictionary with optional keys min_sg, min_core, min_engine and min_desktop
ConfigDescriptor
- class sgtk.descriptor.ConfigDescriptor(sg_connection, io_descriptor, bundle_cache_root_override, fallback_roots)[source]
Descriptor that describes a Toolkit Configuration
Note
Use the factory method
create_descriptor()
when creating new descriptor objects.- Parameters:
sg_connection – Connection to the current site.
io_descriptor – Associated IO descriptor.
bundle_cache_root_override – Override for root path to where downloaded apps are cached.
fallback_roots – List of immutable fallback cache locations where apps will be searched for.
- property associated_core_descriptor
The descriptor dict or url required for this core or
None
if not defined.- Returns:
Core descriptor dict or uri or
None
if not defined
- property python_interpreter
Retrieves the Python interpreter for the current platform from the interpreter files.
Note
Most runtime environments (Nuke, Maya, Houdini, etc.) provide their own python interpreter that needs to used when executing code. This property is useful if the engine you are running (e.g.
tk-shell
) does not have an explicit interpreter associated.- Raises:
TankFileDoesNotExistError
If the interpreter file is missing.- Raises:
TankInvalidInterpreterLocationError
If the interpreter can’t be found on disk.- Returns:
Path value stored in the interpreter file.
- get_associated_core_feature_info(feature_name, default_value=None)[source]
Retrieves information for a given feature in the manifest of the core.
- The
default_value
will be returned in the following cases: a feature is missing from the manifest
the manifest is empty
the manifest is missing
there is no core associated with this configuration.
- The
- property version_constraints
A dictionary with version constraints. The absence of a key indicates that there is no defined constraint. The following keys can be returned: min_sg, min_core, min_engine and min_desktop
- Returns:
Dictionary with optional keys min_sg, min_core, min_engine and min_desktop
- property readme_content
Associated readme content as a list. If not readme exists, an empty list is returned
- Returns:
list of strings
- associated_core_version_less_than(version_str)[source]
Attempt to determine if the associated core version is less than a given version. Returning True means that the associated core version is less than the given one, however returning False does not guarantee that the associated version is higher, it may also be an indication that a version number couldn’t be determined.
- Parameters:
version_str – Version string, e.g. ‘0.18.123’
- Returns:
true if core version is less, false otherwise
- property required_storages
A list of storage names needed for this config. This may be an empty list if the configuration doesn’t make use of the file system.
- Returns:
List of storage names as strings
- property storage_roots
A
StorageRoots
instance for this config descriptor.Returns None if the config does not define any storage roots.
- property changelog
Information about the changelog for this item.
- Returns:
A tuple (changelog_summary, changelog_url). Values may be
None
to indicate that no changelog exists.
- clone_cache(cache_root)
The descriptor system maintains an internal cache where it downloads the payload that is associated with the descriptor. Toolkit supports complex cache setups, where you can specify a series of path where toolkit should go and look for cached items.
This is an advanced method that helps in cases where a user wishes to administer such a setup, allowing a cached payload to be copied from its current location into a new cache structure.
If the descriptor’s payload doesn’t exist on disk, it will be downloaded.
- Parameters:
cache_root – Root point of the cache location to copy to.
- copy(target_folder)
Copy the config descriptor into the specified target location.
- Parameters:
target_folder – Folder to copy the descriptor to
- property deprecation_status
Information about deprecation status.
- Returns:
Returns a tuple (is_deprecated, message) to indicate if this item is deprecated.
- property description
A short description of the item.
- property display_name
The display name for this item. If no display name has been defined, the system name will be returned.
- property documentation_url
The documentation url for this item. If no documentation url has been defined, a url to the toolkit user guide is returned.
- download_local()
Retrieves this version to local repo.
- ensure_local()
Helper method. Ensures that the item is locally available.
- exists_local()
Returns true if this item exists in a local repo.
- find_latest_cached_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version that can be found in the local bundle caches.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
Instance derived from
Descriptor
orNone
if no cached version is available.
- find_latest_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
instance derived from
Descriptor
- get_dict()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_location()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_path()
Returns the path to a location where this item is cached.
When locating the item, any bundle cache fallback paths will first be searched in the order they have been defined, and lastly the main bundle cached will be checked.
If the item is not locally cached,
None
is returned.- Returns:
Path string or
None
if not cached.
- get_uri()
Returns the uri associated with this descriptor The uri is a string based representation that is equivalent to the descriptor dictionary returned by the get_dict() method.
- Returns:
Uri string that can be used to construct the descriptor
- has_remote_access()
Probes if the current descriptor is able to handle remote requests. If this method returns, true, operations such as
download_local()
andfind_latest_version()
can be expected to succeed.- Returns:
True if a remote is accessible, false if not.
- property icon_256
The path to a 256px square png icon file representing this item
- is_dev()
Returns true if this item is intended for development purposes
- Returns:
True if this is a developer item
- is_immutable()
Returns true if this descriptor never changes its content. This is true for most descriptors as they represent a particular version, tag or commit of an item. Examples of non-immutable descriptors include path and dev descriptors, where the descriptor points at a “live” location on disk where a user can make changes at any time.
- Returns:
True if this is a developer item
- property support_url
A url that points at a support web page associated with this item. If not url has been defined,
None
is returned.
- property system_name
A short name, suitable for use in configuration files and for folders on disk.
- property version
The version number string for this item.
CoreDescriptor
- class sgtk.descriptor.CoreDescriptor(sg_connection, io_descriptor, bundle_cache_root_override, fallback_roots)[source]
Descriptor object which describes a Toolkit Core API version.
Note
Use the factory method
create_descriptor()
when creating new descriptor objects.- Parameters:
sg_connection – Connection to the current site.
io_descriptor – Associated IO descriptor.
bundle_cache_root_override – Override for root path to where downloaded apps are cached.
fallback_roots – List of immutable fallback cache locations where apps will be searched for.
- property version_constraints
A dictionary with version constraints. The absence of a key indicates that there is no defined constraint. The following keys can be returned: min_sg, min_core, min_engine and min_desktop
- Returns:
Dictionary with optional keys min_sg, min_core, min_engine, min_desktop
- get_feature_info(feature_name, default_value=None)[source]
Retrieves information for a given feature in the manifest.
- The
default_value
will be returned in the following cases: a feature is missing from the manifest
the manifest is empty
the manifest is missing
- The
- copy(target_folder)[source]
Copy the config descriptor into the specified target location.
- Parameters:
target_folder – Folder to copy the descriptor to
- property changelog
Information about the changelog for this item.
- Returns:
A tuple (changelog_summary, changelog_url). Values may be
None
to indicate that no changelog exists.
- clone_cache(cache_root)
The descriptor system maintains an internal cache where it downloads the payload that is associated with the descriptor. Toolkit supports complex cache setups, where you can specify a series of path where toolkit should go and look for cached items.
This is an advanced method that helps in cases where a user wishes to administer such a setup, allowing a cached payload to be copied from its current location into a new cache structure.
If the descriptor’s payload doesn’t exist on disk, it will be downloaded.
- Parameters:
cache_root – Root point of the cache location to copy to.
- property deprecation_status
Information about deprecation status.
- Returns:
Returns a tuple (is_deprecated, message) to indicate if this item is deprecated.
- property description
A short description of the item.
- property display_name
The display name for this item. If no display name has been defined, the system name will be returned.
- property documentation_url
The documentation url for this item. If no documentation url has been defined, a url to the toolkit user guide is returned.
- download_local()
Retrieves this version to local repo.
- ensure_local()
Helper method. Ensures that the item is locally available.
- exists_local()
Returns true if this item exists in a local repo.
- find_latest_cached_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version that can be found in the local bundle caches.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
Instance derived from
Descriptor
orNone
if no cached version is available.
- find_latest_version(constraint_pattern=None)
Returns a descriptor object that represents the latest version.
Note
Different descriptor types implements this logic differently, but general good practice is to follow the semantic version numbering standard for any versions used in conjunction with toolkit. This ensures that toolkit can track and correctly determine not just the latest version but also apply constraint pattern matching such as looking for the latest version matching the pattern
v1.x.x
. You can read more about semantic versioning here: http://semver.org/- Parameters:
constraint_pattern –
If this is specified, the query will be constrained by the given pattern. Version patterns are on the following forms:
v0.1.2, v0.12.3.2, v0.1.3beta - a specific version
v0.12.x - get the highest v0.12 version
v1.x.x - get the highest v1 version
- Returns:
instance derived from
Descriptor
- get_dict()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_location()
Returns the dictionary associated with this descriptor
- Returns:
Dictionary that can be used to construct the descriptor
- get_path()
Returns the path to a location where this item is cached.
When locating the item, any bundle cache fallback paths will first be searched in the order they have been defined, and lastly the main bundle cached will be checked.
If the item is not locally cached,
None
is returned.- Returns:
Path string or
None
if not cached.
- get_uri()
Returns the uri associated with this descriptor The uri is a string based representation that is equivalent to the descriptor dictionary returned by the get_dict() method.
- Returns:
Uri string that can be used to construct the descriptor
- has_remote_access()
Probes if the current descriptor is able to handle remote requests. If this method returns, true, operations such as
download_local()
andfind_latest_version()
can be expected to succeed.- Returns:
True if a remote is accessible, false if not.
- property icon_256
The path to a 256px square png icon file representing this item
- is_dev()
Returns true if this item is intended for development purposes
- Returns:
True if this is a developer item
- is_immutable()
Returns true if this descriptor never changes its content. This is true for most descriptors as they represent a particular version, tag or commit of an item. Examples of non-immutable descriptors include path and dev descriptors, where the descriptor points at a “live” location on disk where a user can make changes at any time.
- Returns:
True if this is a developer item
- property support_url
A url that points at a support web page associated with this item. If not url has been defined,
None
is returned.
- property system_name
A short name, suitable for use in configuration files and for folders on disk.
- property version
The version number string for this item.
Exceptions
- class sgtk.descriptor.TankDescriptorError[source]
Bases:
TankError
Base class for all descriptor related errors.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class sgtk.descriptor.TankAppStoreError[source]
Bases:
TankDescriptorError
Errors relating to the Toolkit App Store app store.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class sgtk.descriptor.TankAppStoreConnectionError[source]
Bases:
TankAppStoreError
Errors indicating an error connecting to the Toolkit App Store.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class sgtk.descriptor.TankInvalidAppStoreCredentialsError[source]
Bases:
TankAppStoreConnectionError
Error indicating no credentials for the Toolkit App Store were found in Shotgun.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class sgtk.descriptor.TankCheckVersionConstraintsError(reasons)[source]
Bases:
TankDescriptorError
Error throw when one or more version constraints checks failed.
- property reasons
List of strings explaining why the constraints check failed.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class sgtk.descriptor.TankInvalidInterpreterLocationError[source]
Bases:
TankDescriptorError
Exception that indicates that the interpreter specified in a file was not found.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class sgtk.descriptor.TankMissingManifestError[source]
Bases:
TankDescriptorError
Exception that indicates that the manifest file is missing.
- with_traceback()
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.